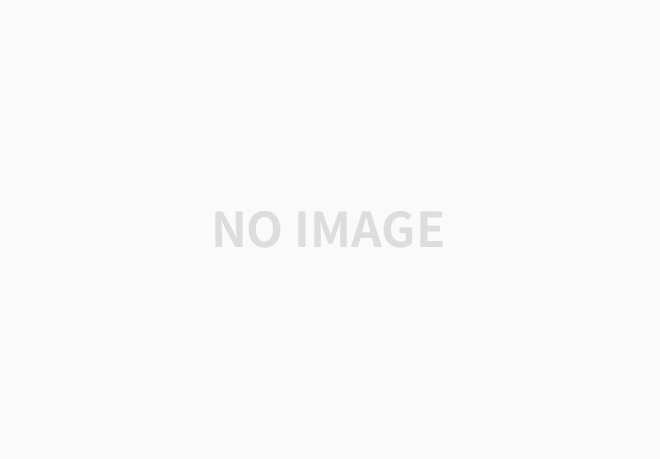
문제 설명
You are given a 2D integer array items
where items[i] = [$price_i$, $beauty_i$] denotes the price and beauty of an item respectively.
You are also given a 0-indexed integer array queries
. For each queries[j]
, you want to determine the maximum beauty of an item whose price is less than or equal to queries[j]
. If no such item exists, then the answer to this query is 0
.
Return an array answer
of the same length as queries
where answer[j]
is the answer to the $j^{th}$ query.
문제 예제
Example 1:
Input: items = [[1,2],[3,2],[2,4],[5,6],[3,5]], queries = [1,2,3,4,5,6]
Output: [2,4,5,5,6,6]
Explanation:
- For queries[0]=1, [1,2] is the only item which has price <= 1. Hence, the answer for this query is 2.
- For queries[1]=2, the items which can be considered are [1,2] and [2,4].
The maximum beauty among them is 4. - For queries[2]=3 and queries[3]=4, the items which can be considered are [1,2], [3,2], [2,4], and [3,5].
The maximum beauty among them is 5. - For queries[4]=5 and queries[5]=6, all items can be considered.
Hence, the answer for them is the maximum beauty of all items, i.e., 6.
Example 2:
Input: items = [[1,2],[1,2],[1,3],[1,4]], queries = [1]
Output: [4]
Explanation:
The price of every item is equal to 1, so we choose the item with the maximum beauty 4.
Note that multiple items can have the same price and/or beauty.
Example 3:
Input: items = [[10,1000]], queries = [5]
Output: [0]
Explanation:
No item has a price less than or equal to 5, so no item can be chosen.
Hence, the answer to the query is 0.
제한 사항
1 <= items.length, queries.length <= 105
items[i].length == 2
1 <= pricei, beautyi, queries[j] <= 109
내 풀이
class Solution:
def maximumBeauty(self, items: List[List[int]], queries: List[int]) -> List[int]:
items.sort(key = lambda x:x[1], reverse = True)
answer = []
for query in queries:
check = False
for p, b in items:
if p <= query:
check = True
answer.append(b)
break
if not check:
answer.append(0)
return answer
이 문제는 items의 각 price와 queries의 각 요소를 비교하여 $price_i$ <= queries[j]를 만족하면서 $beauty_i$가 최대인 값을 찾으면 되는 문제였다.
따라서, 처음에 items를 beauty를 기준으로 내림차순 정렬을 시켜주었다.
- ex)
[[1,2],[3,2],[2,4],[5,6],[3,5]]
---->[[5,6],[3,5],[2,4],[3,2],[1,2]]
그리고 queries의 각 요소와 items의 각 price를 비교하면서 처음 $price_i$ <= queries[j]를 만족하면 $beauty_i$를 바로 answer에 넣어주고 다음 queries의 요소로 넘어가서 앞선 방법을 반복했다.
beauty를 기준으로 정렬했기 때문에 맨 처음 $price_i$ <= queries[j]를 만족하면 그게 max_beauty라 판단한 것이다.
모든 queries의 요소를 돌았음에도 답이 없으면 answer에 0을 넣어주고 답을 맞출 수 있었다.
문제를 보자마자 떠오른 방법은 위에 써놓은 방법인데 문제를 풀고 다른 사람들의 풀이를 보니 이 문제는 이진탐색을 사용하여 푸는 문제인 것 같다. 확실히 내 방법은 input의 길이가 늘어나면 시간이 오래 걸릴 것 같긴하다.
이진탐색을 이용한 풀이
def binary_search(items, q):
left, right = 0, len(items)
while left < right:
mid = (left+right)//2
if items[mid][0] <= q:
left = mid+1
else:
right = mid
return left
class Solution:
def maximumBeauty(self, items: List[List[int]], queries: List[int]) -> List[int]:
items.sort(key = lambda x:(x[0], x[1]))
max_beauty, now_max_beauty = [0], 0
for price, beauty in items:
now_max_beauty = max(now_max_beauty, beauty)
max_beauty.append(now_max_beauty)
answer = []
for q in queries:
answer.append(max_beauty[binary_search(items, q)])
return answer
이와 같이 이진탐색을 사용하여 더 효율적으로 문제를 해결할 수 있다.
문제를 풀기전에 효율적으로 짤 수 있는 또 다른 방법에 대해 더 생각해봐야겠다..!
'알고리즘 > LeetCode' 카테고리의 다른 글
[LeetCode] 2461. Maximum Sum of Distinct Subarrays With Length K - Python (0) | 2024.11.19 |
---|---|
[LeetCode] 1652. Defuse the Bomb - Python (0) | 2024.11.18 |
[LeetCode] 2064. Minimized Maximum of Products Distributed to Any Store - Python (1) | 2024.11.14 |
[LeetCode] 2563. Count the Number of Fair Pairs - Python (1) | 2024.11.13 |
[LeetCode] 2601. Prime Subtraction Operation - Python (2) | 2024.11.12 |